You can set the RSTDISBL (bit 7 on the HIGH fuse) to disable the external reset and use the reset pin as PC6 to manage the reset behavior from your program.
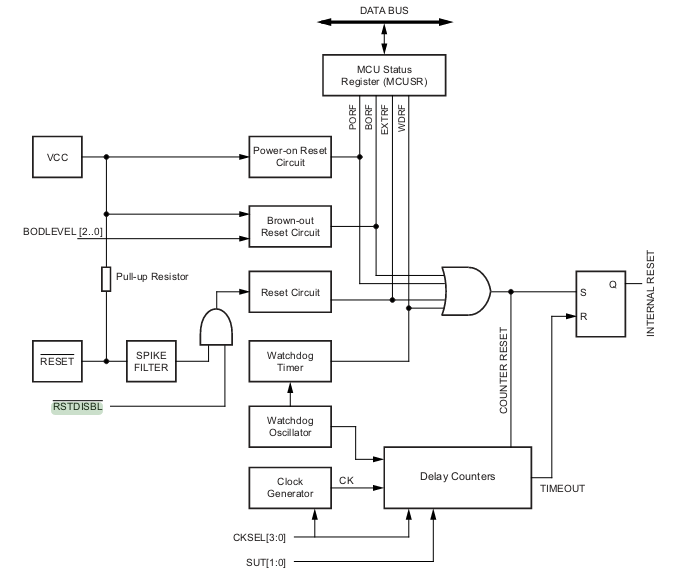
The second part is to capture when reset button is pressed: put some code in the ISR for PCINT1 handler when the pin changes (PCINT14 pin function). If you are programming an Arduino board, the reset button is pulled up (press becomes LOW and release becomes HIGH).
Detecting long press or short press is a timer affair. According to your requirements, you will restore some EEPROM values or external state to factory defaults before the microcontroller resets with a long press.
This C snippet will reset the microcontroller after the short or long press (watchdog timer feature):
#include <avr/wdt.h>
// ...
wdt_enable(WDTO_15MS);
for (;;) {}