So you leds are connected to "pins" not "ports." Since each of the buttons will turn on only 1 led we need to keep track of which button pairs with which led. To do this we can use a struct
like this one:
struct ButtonLed{
int buttonPin;
int ledPin;
};
You declare a ButtonLed
like this: ButtonLed button1 = {1, 0}
where the first parameter is the button's pin and the second is the led's pin. (All of the led pins are set to 0 since I don't know which pins you have them connected to.)
And the array will hold the button/led pin pair like this:
ButtonLed questionNumberArray[] = {button1, button2, button3, button4, button5, button6};
Since there are 6 leds you know that your array is size 6 so use this declaration instead
const int n = 6;
I fixed you range for the random number generation. Look at the documentation for random()
here https://www.arduino.cc/en/Reference/Random. We can use this function for shuffling the array.
void shuffleArray (ButtonLed arr [], const int size){
for (int i = 0; i < size - 1; i++){ // iterates through array except last element
int j = random(0, size); // generates a random index from (0 to n-1) inclusive
// swap current index with random index
ButtonLed t = arr[i];
arr[i] = arr[j];
arr[j] = t;
}
}
We will use this function to wait until the user enters a sequence of button presses:
ButtonLed getButtonPress (ButtonLed arr [], const int size){
bool pressed = false;
ButtonLed pressedButton;
while (!pressed){ // loops until a button was pressed
for (int i = 0; i < size && !pressed; i++){ // !pressed allows early out
if (digitalRead(arr[i].buttonPin)){ // one of the six buttons was pressed
pressed = true; // exit both loops
pressedButton = arr[i]; // stores which button was pressed
}
}
}
return pressedButton; // return the pressed button
}
Essentially it gets stuck in a loop and reads each of the 6 buttons until one of them is pressed and then records which one was pressed.
Try using the code below and read the comments the only thing you need to do is change all the zeros in the ButtonLed
declarations to whatever pin the leds are connected to. Also the struct
above needs to go into it's only file called "ButtonLed.h" It must be called that.
#include "ButtonLed.h"
// These are ButtonLeds our newly created data type
// they hold both an led's pin and the pin for the button
// that turns it on
ButtonLed button1 = {1, 10};
ButtonLed button2 = {2, 8};
ButtonLed button3 = {9, 7};
ButtonLed button4 = {11, 6};
ButtonLed button5 = {12, 5};
ButtonLed button6 = {13, 4};
// This function will randomly shuffle an array of ButtonLeds
void shuffleArray (ButtonLed arr [], const int size){
for (int i = 0; i < size - 1; i++){ // iterates through array except last element
int j = random(0, size); // generates a random index from (0 to size-1) inclusive
// swap current index with random index
ButtonLed t = arr[i];
arr[i] = arr[j];
arr[j] = t;
}
}
ButtonLed getButtonPress (ButtonLed arr [], const int size){
bool pressed = false;
ButtonLed pressedButton;
while (!pressed){ // loops until a button was pressed
for (int i = 0; i < size && !pressed; i++){ // !pressed allows early out
if (digitalRead(arr[i].buttonPin)){ // one of the six buttons was pressed
digitalWrite(digitalRead(arr[i].ledPin, HIGH); // turns on the led the user chose
delay(200);
digitalWrite(digitalRead(arr[i].ledPin, LOW); // turns it back off
pressed = true; // exit both loops
pressedButton = arr[i]; // stores which button was pressed
}
}
}
return pressedButton; // return the pressed button
}
void setup() {
randomSeed(analogRead(A0));
// This is how you set up the leds for output
pinMode(button1.ledPin, OUTPUT);
pinMode(button2.ledPin, OUTPUT);
pinMode(button3.ledPin, OUTPUT);
pinMode(button4.ledPin, OUTPUT);
pinMode(button5.ledPin, OUTPUT);
pinMode(button6.ledPin, OUTPUT);
// This is how you set up the buttons for input
pinMode(button1.buttonPin, INPUT);
pinMode(button2.buttonPin, INPUT);
pinMode(button3.buttonPin, INPUT);
pinMode(button4.buttonPin, INPUT);
pinMode(button5.buttonPin, INPUT);
pinMode(button6.buttonPin, INPUT);
}
void loop() {
// This array is of type ButtonLed our newly created data type
ButtonLed questionNumberArray[] = {button1, button2, button3,
button4, button5, button6};
const size_t n = 6; // array's size
shuffleArray(questionNumberArray, n);
numLeds = 1;
bool guessCorrectly = false;
// this while loop allows the user to keep trying until they get it right
while (!guessCorrectly){
// This loop displays the correct sequence to the user
for (int i = 0; i < numLeds; i++){
digitalWrite(questionNumberArray[i].ledPin, HIGH); // turn on the led
delay(500); // decrease this number to flash faster; increase to flash slower
digitalWrite(questionNumberArray[i].ledPin, LOW); // turn off the led
}
bool correctButton = true; // the user has pressed the correct button
ButtonLed userSequence [6]; // stores the sequence the user entered
// lets the user push 6 buttons
for (int i = 0; i < numLeds; i++){
userSequence[i] = getButtonPress(questionNumberArray, numLeds);
delay(500); // delay between button presses
}
// check if the user entered the correct sequence
for (int i = 0; i < numLeds && correctButton; i++){
if (userSequence[i].buttonPin != questionNumberArray[i].buttonPin)
correctButton = false;
}
if (correctButton){ // user entered the correct sequence
guessCorrectly = true;
// This will turn on the leds in the correct sequence
for (int i = 0; i < numLeds; i++){
digitalWrite(questionNumberArray[i].ledPin, HIGH); // turn on the led
delay(500); // delay half a second
digitalWrite(questionNumberArray[i].ledPin, LOW); // turn off the led
}
numLeds++;
if (numLeds > 6)
--numLeds;
}
}
}
After you are finished typing the code above it ctrl+shift+n
and open a new tab called "ButtonLed.h" and add this to it:
#ifndef BUTTONLED_H
#define BUTTONLED_H
#include <Arduino.h>
// We create new data type called ButtonLed which stores the button's pin
// and the led's pin; this allows us to keep both an led and it's
// corresponding button together without have to manage 2 arrays
struct ButtonLed{
int buttonPin;
int ledPin;
};
#endif
Make sure you open a new tab and not a new project. Also as others have said you should really learn how to program. Be aware this code compiles however, I haven't tested it. I will update the code when you tell me what pins the leds are connected to.
Edit 1
I added a while loop that allows the user to keep trying until they guess correctly.
Edit 2
I also updated the code for which pins the leds are connected to. Remember they're connected to "pins" Not "ports.
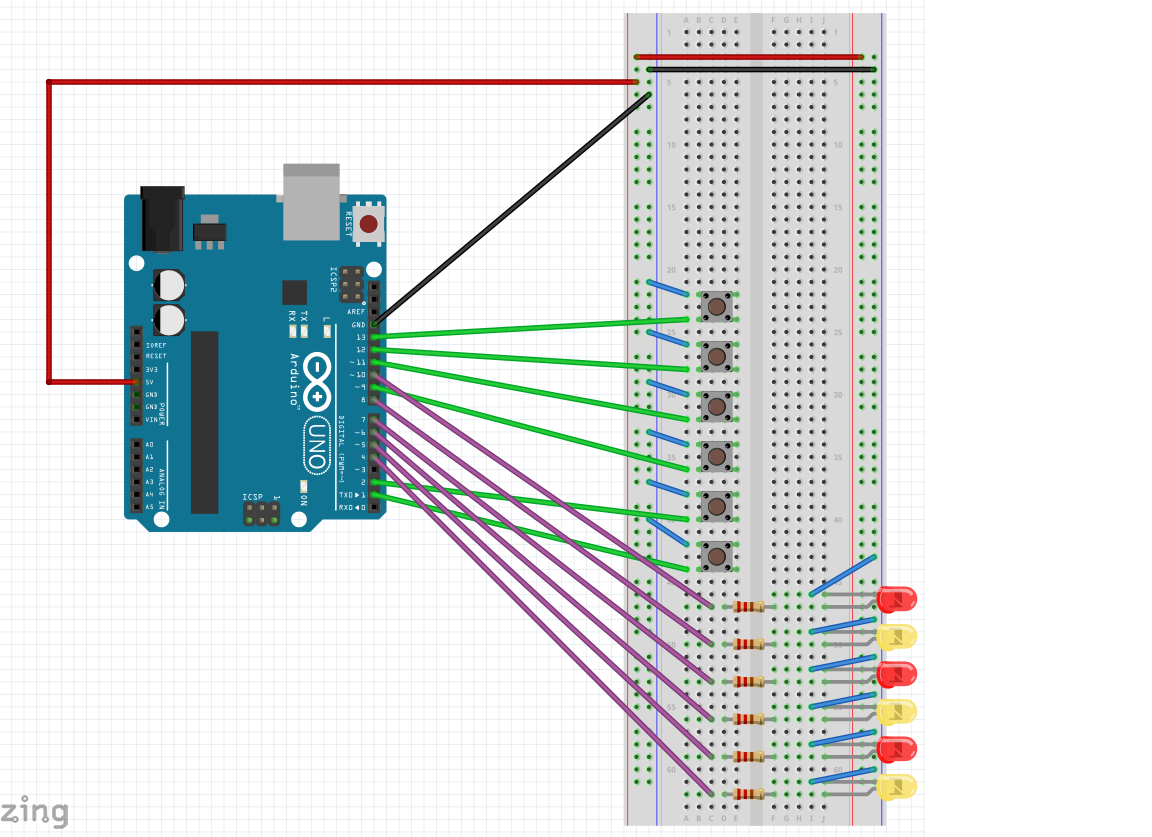