Instead of printing '0' or '1' set the LED pin off or on, like this:
const int LEDTrans = 13;
const int timeWaitOn = 1000; // millisec (1 sec)
const int timeWaitOff = 1000; // millisec (1 sec)
void setup() {
// Turn the Serial Protocol ON
Serial.begin(115200);
Serial.println("Send letter:");
pinMode (LEDTrans, OUTPUT);
}
void loop() {
/* check if data has been sent from the computer: */
if (Serial.available()) {
byte byteRead = Serial.read();
// will print the binary representation of 'byteRead' as 8 characters of '1's and '0's, MSB first.
for ( uint8_t bitMask = 128; bitMask != 0; bitMask >>= 1 ) {
if ( byteRead & bitMask ) {
digitalWrite(LEDTrans, HIGH); //turn the led on
delay(timeWaitOn); //delay in millisec (1 sec)
digitalWrite(LEDTrans, LOW); //turn the led off
} else {
delay(timeWaitOff); //wait a sec
} // end of if
} // end of for loop
} // end of if something available
} // end of loop
how do i count the flashes? is it based on the binary 01000001?
Here's the problem ... according to what your question seems to ask for, you are wanting an LED to flash for a 1 and be off for a zero. So the results (which my code provides) would look like this:
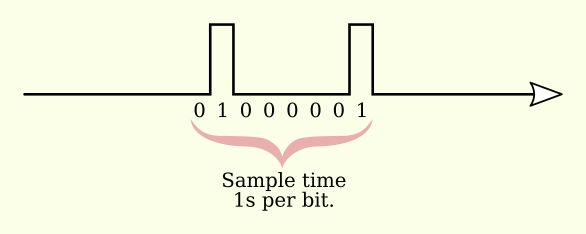
So, you get two flashes, the first one second after it starts, then there is a gap of 5 seconds, and then another flash. That would be 01000001.
However, how do you know when to start counting flashes? It might be:
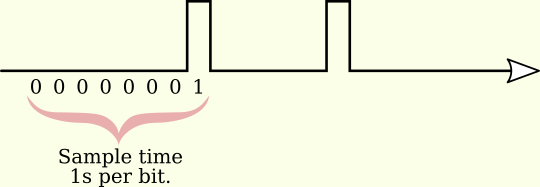
That would be 00000001.
Or even:
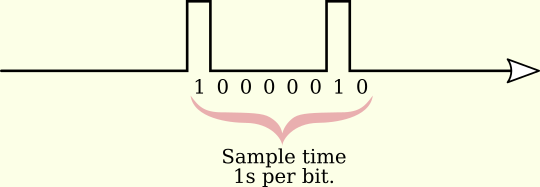
That would be 10000010.
You can see, can't you, that you can't make any meaningful deduction at the receiving end about what the character is?
So what asynchronous serial (normal serial on an Arduino) does is have a Start Bit which signals when to start counting, like this:
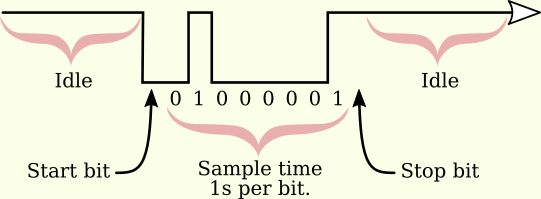
Normally the serial port is high (continuous 1s) and then when a 0 arrives that means "start counting". So, you wait 1½ bits of time and then sample at the known baud rate. In your example, where you want 1 second per bit, the baud rate would be 1 (one bit per second). Thus, you sample every one second. Normally baud rates are somewhat faster than that, like 9600 or 115200 samples per second.
Also there is a Stop Bit which is always a 1. This guarantees that there will be at least a single 1 at the end of each byte, so that we can tell when the next start bit arrives.
There are other ways of doing this, for example a short pulse might represent a zero, and a longer pulse represent a one. In that case, any pulse at all could represent the start of a byte, and then you just measure how many short pulses, and how many long pulses, you get (for some definition of "short" and "long").